PHP面试题整理
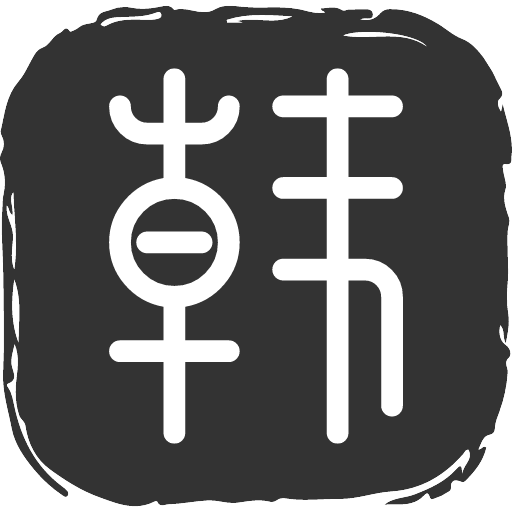
常见的面试题整理,来源是各个地方…
基础篇
1. 表单提交中的Get和Post的异同点
1 | 语义不同: |
2. 用PHP写出显示客户端IP与服务器IP的代码
1 | // 使用超全局变量$_SERVER |
3. include和require的区别是什么?
1 | 两个函数都是包含引入的文件 |
4. PHP 不使用第三个变量实现交换两个变量的值
1 | $a = 1; |
5. 取文件的扩展名
1 | function getExt1($fileName) |
6. 用PHP header()函数实现页面404错误提示功能
1 | Header("HTTP/1.1 404 Not Found"); |
7. 约瑟夫环 n 只猴子,数m踢出一只,最后的就是大王
1 | function king($n, $m) |
8. 文件加锁写入
1 | // w+ : 读写方式打开 |
9. 遍历文件夹
1 | function myDir($path) |
10. 单例模式
1 | class DB |